What is XSS?
February 14, 2023
XSS (Cross-Site Scripting) is a common type of web attack. As a front-end or back-end engineer, you are likely to be asked about XSS in an interview, as it is important for you to have a basic understanding of the concept.
What is XSS?
An XSS attack occurs when a malicious user injects an attack script from the client-side to achieve a certain goal, such as stealing cookies, sessions, passwords, and so on, which in turn affects other users. The reason why it is called cross-site is that this type of attack is usually initiated from a trusted source, and therefore can bypass the same-origin policy. For example, when a user enters a legitimate website with a legitimate identity, the website considers the input to be trustworthy. However, when this user injects an attack script, the website also considers the script to be trustworthy.
The most common XSS attack is injecting a JavaScript script into an input field. For example, a malicious user injects an attack script into an input box on a forum website, which becomes a forum post. When other users visit the forum and view the post with the malicious script, they become vulnerable to the attack.
Users can enter malicious JavaScript syntax to cause problems with the system. You can try this on this website if you are interested.
Since JavaScript can do almost anything, XSS attacks can take many forms. For example, they can steal other users' cookies and use them to send requests or alter website content. Here is an example of a prank that was made possible through an XSS attack on the live streaming platform Twitch.
XSS Attack Types
Stored XSS
If the malicious script is stored in the database, it is called Stored XSS. The most common example is articles, comments, etc., because users can enter content arbitrarily. If it is not checked, <script>
tags will be treated as normal HTML for execution.
Reflected XSS
This type of XSS attack is reflected in the response from the server. This type of XSS attack is not stored in the database, but is mainly triggered by malicious requests from users. If the backend does not filter and directly returns the result to the front end, it is possible to execute malicious code. For example:
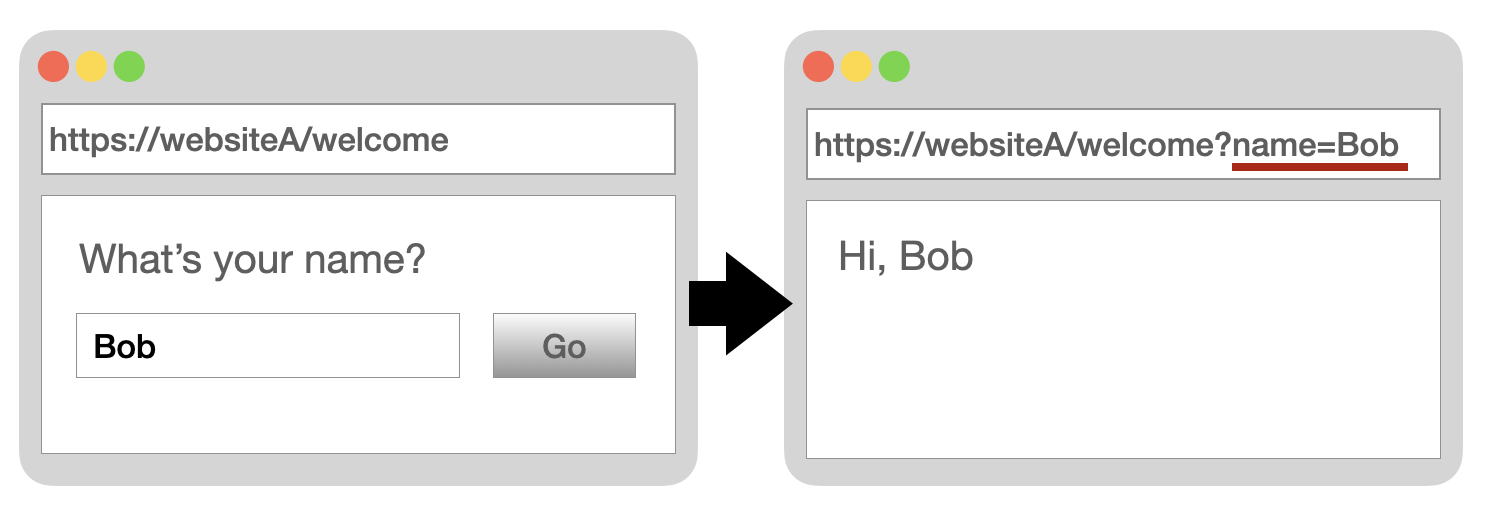
When the user fills in the name, the following code is displayed:
<h3> Hi, <?=$_GET['name'] ?> </h3>
The user's input is likely to be maliciously filled in as:
https://websiteA/welcome?name=<script>alert(123)</script>
This method is usually used to lure users into clicking links through phishing, social engineering, etc.
DOM-Based XSS
DOM stands for Document Object Model. It can dynamically generate a complete web page through Javascript without going through the backend. Therefore, DOM-Based XSS refers to Javascript on the web page that does not check the input data during execution, which causes the operation DOM to carry malicious code.
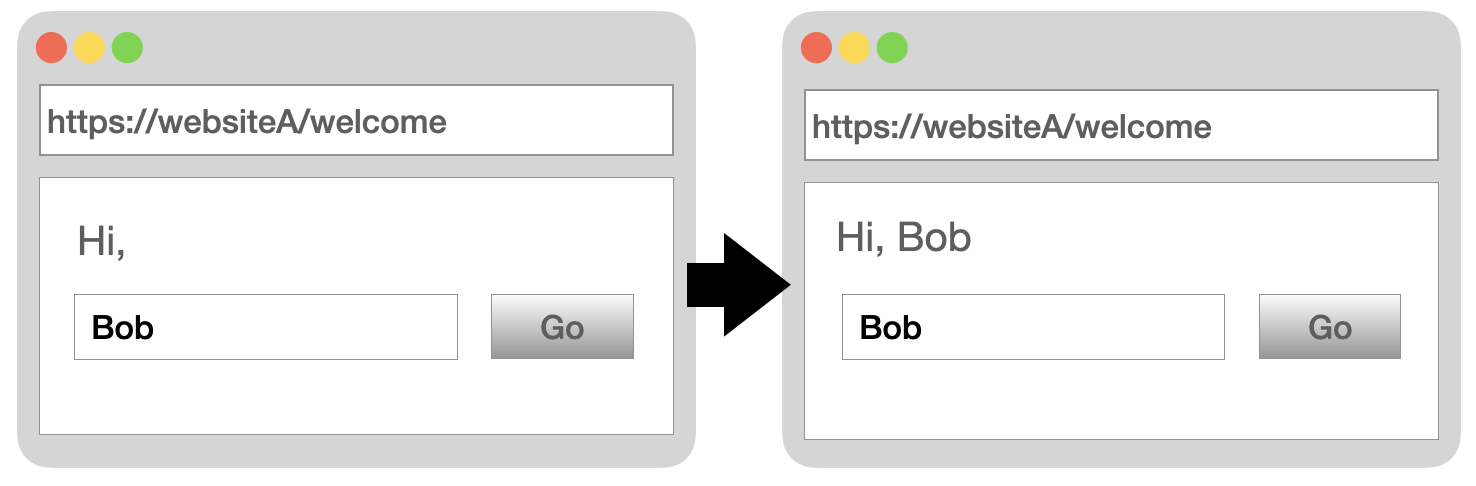
In the same URL, the content is displayed directly on the front-end page through DOM.
<script>
var hello = function () {
let name = document.getElementById("name").value;
document.getElementById("show").innerHTML = name;
};
</script>
<h3>Hi, <span id="show"></span></h3>
<input id="name" type="text" />
<button onclick="hello();">Go</button>
As a result, if the input content is written as
<img src="#" onerror="alert(123);" />
The browser will try to load the image, but since the image source is #
, the browser will not be able to load the image, triggering onerror
, and executing alert(123)
.
This type of attack may not be able to directly enter the syntax, but can be combined with Stored XSS and Reflected XSS to make content, and then use Javascript to dynamically generate valid DOM objects to execute malicious code.
How To Defend Against XSS?
DOM-Based Prevention - Check input fields. This attack needs to be prevented from the front-end. Any input field, such as comment fields, file upload fields, form fields, etc., should have an escape mechanism that converts scripts into strings. For example, if an attacker enters
<script>alert(1)</script>
, we convert<
to<
, and it will still appear as<
on the screen, but it will not be parsed as an HTML tag by the program.Ensure that user-generated scripts are not executed. Use CSP (content security policy) to set which domains scripts should be executed from, and the browser will only execute those scripts. For example, if only scripts from the same domain as the website are allowed to be executed, other scripts injected by malicious attackers will be recognized as not to be executed and will not be executed.
Stored and Reflected Prevention. Both of these must be prevented by the back-end. Any user input that is allowed must be checked, and related keywords, such as
<script>
andonerror
commands, must be deleted.