How to Refactor? What Are the Benefits of Refactoring?
March 3, 2023
What is refactoring?
Refactoring is the process of "restructuring code without changing its external behavior". Therefore, for external users, refactoring is not noticeable, but the benefits of refactoring are:
- Improved readability: Through refactoring, the code becomes clearer, concise, and better organized, thus improving readability and understanding.
- Improved maintainability: Refactored code is easier to understand and modify, which helps with maintenance.
- Reduced redundancy: Refactoring can remove unused code, consolidate duplicate code, and reorganize code, thereby reducing code redundancy.
- Improved performance: Refactoring can help improve code performance, such as by reducing the number of loops and using more efficient data structures.
- Improved security: Refactoring can remove code that is vulnerable to attacks, improving the security of the code.
When should you refactor?
Refactoring is an ongoing process, and it is suitable to start refactoring when the following situations occur:
- Code duplication: Refactoring can eliminate duplicate code, making the code library more concise.
- Technical debt: Over time, the code library may become messy and difficult to maintain, leading to the accumulation of technical debt. Refactoring can reduce this debt by improving code structure and organization.
- Adding new features: When adding new features to existing code, refactoring can ensure that the code remains maintainable and scalable.
- Performance optimization: If the code runs too slowly or requires too many resources, refactoring can improve performance by addressing bottlenecks and inefficiencies.
- Bug fixing: Refactoring can make it easier to find and fix errors in the code while preventing new errors from being introduced.
- Team collaboration: If multiple developers are working on the same code library, refactoring can improve collaboration by making the code easier to understand and maintain.
How to refactor?
Good refactoring advice is to make small changes that do not affect external behavior. Here are some common techniques:
Red-Green-Refactor
Red-Green-Refactor is a software development process that is usually used for test-driven development (TDD). This process is divided into three steps:
- Red: Developers first write a failing test to verify that the required functionality does not exist or is incorrect.
- Green: Developers then write the minimum amount of code to pass the test.
- Refactor: Developers review the code again to ensure that its structure is correct, maintainable, and easy to understand.
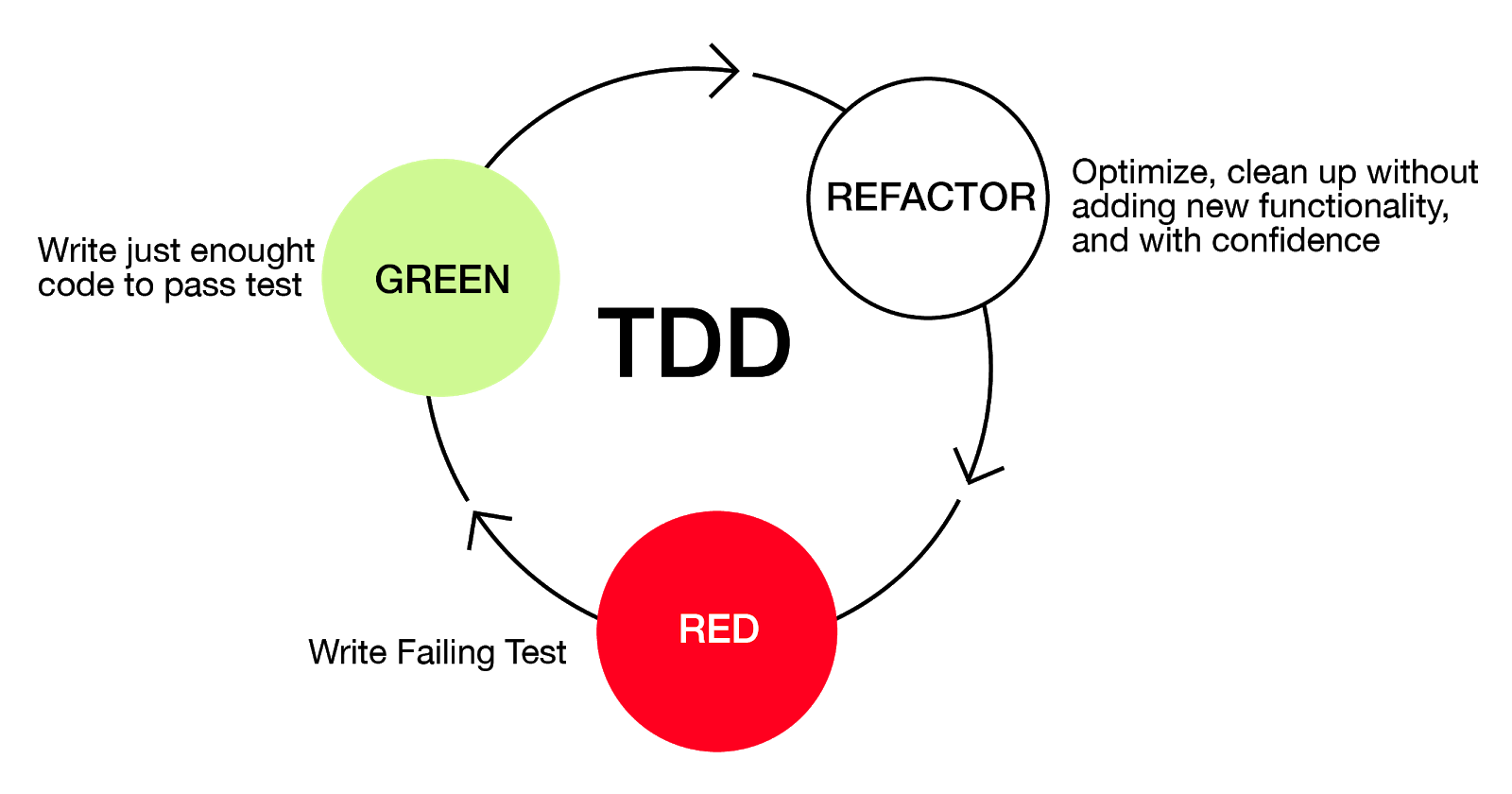
As shown in the figure above, this way, developers can ensure that the code meets standards and avoid confusion caused by complex code. This method helps ensure code quality and ensures program correctness by frequent testing.
Abstraction
If there are many places that need to be refactored, abstraction can be used to do class inheritance, hierarchical structure, and extraction to reduce duplicate and unnecessary code.
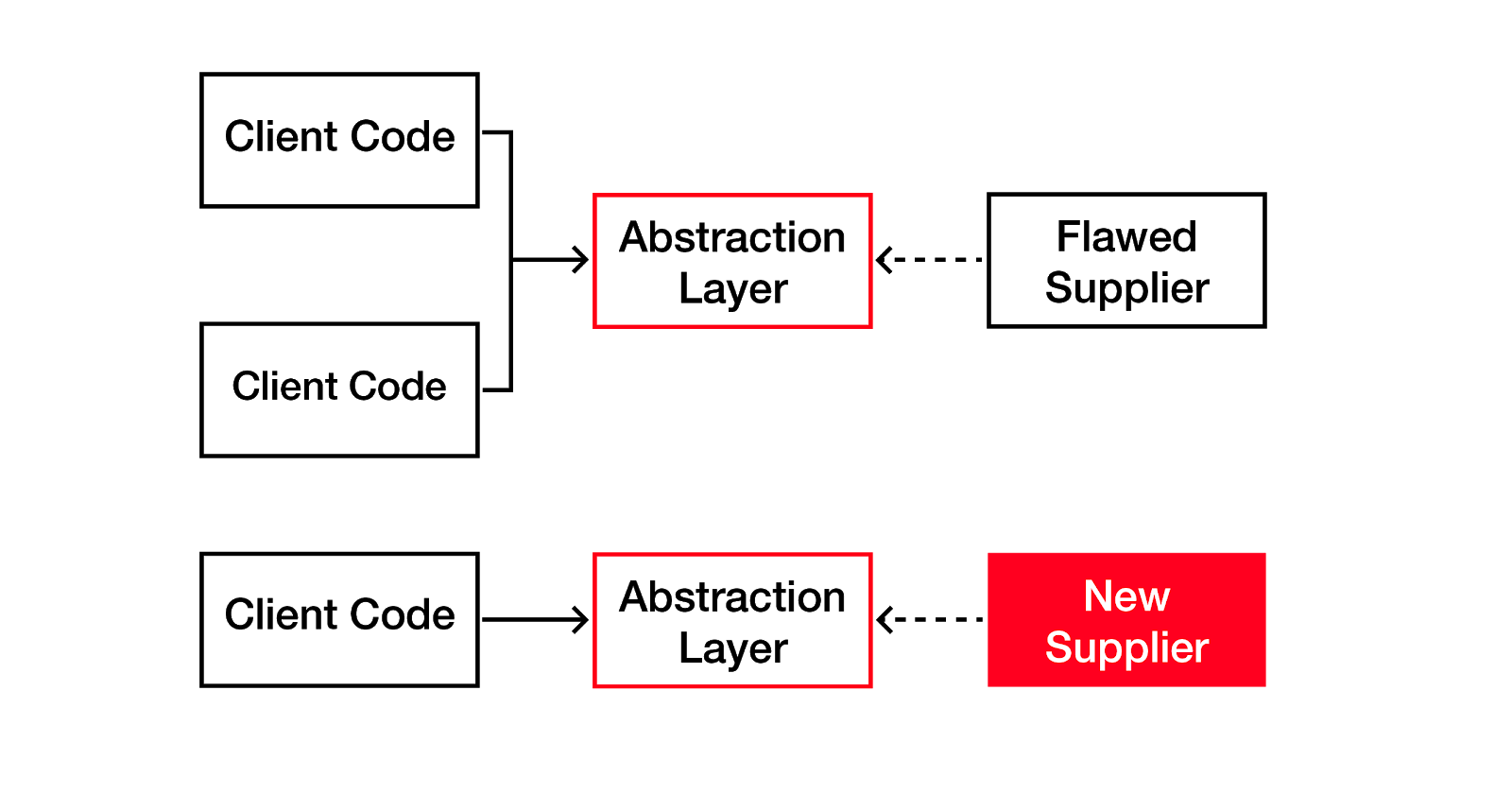
The above figure shows the Pull-Up and Push-Down methods. Pull-Up moves methods from a subclass to its superclass, while Push-Down moves methods from a superclass to its subclass.
Composing Method
- Extract Method: If a piece of code can be isolated, it can be placed in a separate function with a name that explains its purpose. There are examples of refactoring before and after below.
The original code is:
void Process(MyDataSet mds) {
int result = 0;
if (mds.isReady) {
int data1 = mds.param[0];
int data2 = mds.param[1];
result = data1 % data2;
}
}
The refactored code is:
void Process(MyDataSet mds){
int result = 0;
if (mds.isReady)
result = CalculateMDS(mds.param[0], mds.param[1]);
}
int CalculateMDS(int data1, int data2) {
return data1 % data2;
}
It is possible to place less understandable code into a new method and give it a meaningful name, which can improve readability and maintainability.
- Inline Method: In the place where the function is called, insert the function body directly and remove the function. There are examples of refactoring before and after below.
The original code is:
class PizzaDelivery {
// ...
int getRating() {
return moreThanFiveLateDeliveries() ? 2 : 1;
}
boolean moreThanFiveLateDeliveries() {
return numberOfLateDeliveries > 5;
}
}
The refactored code is:
class PizzaDelivery {
// ...
int getRating() {
return numberOfLateDeliveries > 5 ? 2 : 1;
}
}
If some of the code in a function is just as clear and understandable as the function name, the function can be removed.